Mindbreeze only allows the user to find objects for which they have access rights for. The authorization of objects can be carried out with Access Control Lists (ACLs) and Live Access Check. ACLs are preferred because they are quicker.
ACLs
A list of principals can be saved for each object. During a search a check is performed to see whether the principal of the logged in user is currently part of this list. The corresponding ACL entry then decides whether the object can be found or not. Typically user and group names are used as principals. A typical ACL looks as follows:
john doe:GRANT, marketing:GRANT
This gives the user john doe and the group marketing access to the object. All other principals are unable to find the object.
The order can be used to structure the ACLs. The ACL
john doe:DENY, marketing:GRANT
allows all marketing group members except john doe access. The result is the value of the first coinciding principals. Conversely, the ACL
marketing:GRANT, john doe:DENY
allows john doe access to the document, even if he is a member of the marketing group.
The ACLs are set with setAcl
during indexing:
indexable.setAcl(AccessControlList.newBuilder() .addEntry(AccessControlEntry.newBuilder() .setPrincipal("john doe") .setAction(AccessControlEntry.Action.GRANT) ).build() );
The assignment of principals to the logged in user is decisive for the checking of the ACLs. The resolveUser
method, which is also part of the plug-in, serves this purpose.
<Plugin> … <Extension> <point>com.mindbreeze.query.mes3.Authorization</point> </Extension> … <java_class>mindbreeze.example.cmis.query.CmisAuthorizationProvider</java_class> … </Plugin>
The following example only shows the assignment of a group with a string comparison. For a specific data source the principals are assigned from the data source or via e.g. Microsoft Active Directory.
public Collection<String> resolveUser(Principal principal, String categoryInstance) { LinkedList<String> principals = new LinkedList<String>(); principals.add(principal.getName().toLowerCase()); if ("john doe".equalsIgnoreCase(principal.getName())) { principals.add("marketing"); } return principals; }
The concept of principals is important because changes to group memberships don’t therefore require an updating of all objects in the index. The principals from resolveUser just need to be correctly delivered and the results are displayed correctly.
For authorisation using ACLs Mindbreeze provides the following settings.
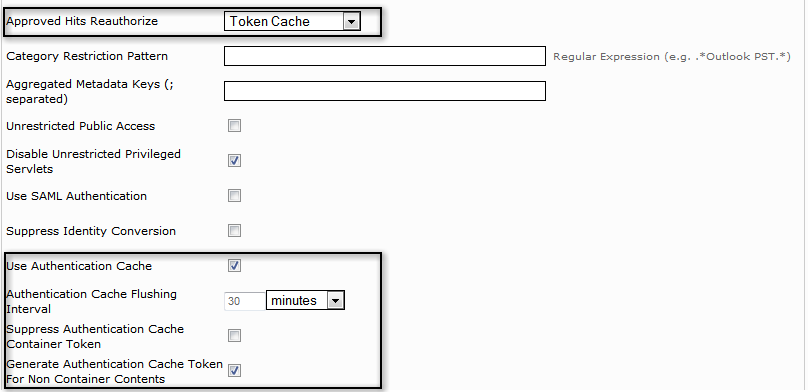
The “Use Authentication Cache” option is activated by default and allows the caching of cacheable authentication results within an “Authentication Cache Flushing Interval”.
Live access check
Warning: Live Access Check can severely reduce the search speed!
For Live Access Check all found documents are transmitted to the authorize
method of the AuthorizationPlugin. This method decides for each document whether the user is allowed to find the document or not.
public void authorize(AuthorizationRequest request) { boolean isJohnDoe = "john doe".equalsIgnoreCase(request.getPrincipal().getName()); for (AuthorizableObject authorizableObject : request.getObjects()) { if (isJohnDoe && authorizableObject.getKey().contains("john doe")) { authorizableObject.authorize(); } else { authorizableObject.reject(); } } }
This example only shows a string comparison. For a specific data source the data source is asked whether the user is allowed to see the object.
The advantage of this approach is that the result of the access rights check is always up to date. However this is at the cost of speed as ACLs can be checked much quicker than an inquiry can be sent to a data source.
One possibility is a two-step process where first of all the ACLs are checked and then a Live Access Check is carried out for those remaining results. This ensures that the user cannot see any documents for which they no longer have access rights where the ACLs haven’t yet been updated.
If External Authorizer is selected in the "Approved Hits Reauthorize" dropdown box then the ACLs aren’t checked.
Download
Access the Mindbreeze InSpire SDK download area.